Data Structure and Algorithm
Basic Introduction
Unit 1:Concept and definition of data structures
• Information and its meaning
• Array in C
• The array as an ADT
• One dimensional array
• Two dimensional array
• Multidimensional array
• Structure
• Union
• Pointer
What is data structure?
- Data structure is a way of organizing all data items and establishing relationship among those data items.
- Data structures are the building blocks of a program.
Data structure mainly specifies the following four things:
• Organization of data.
• Accessing methods
• Degree of associativity
• Processing alternatives for information
To develop a program of an algorithm, we should select an appropriate data structure for that algorithm. Therefore algorithm and its associated data structures form a program. Algorithm + Data structure = Program
A static data structure is one whose capacity is fixed at creation. For example, array. A dynamic data structure is one whose capacity is variable, so it can expand or contract at any time. For example, linked list, binary tree etc.
Abstract Data Types (ADTs)
An abstract data type is a data type whose representation is hidden from, and of no concern to the application code. For example, when writing application code, we don’t care how strings are represented: we just declare variables of type String, and manipulate them by using string operations.
Once an abstract data type has been designed, the programmer responsible for implementing that type is concerned only with choosing a suitable data structure and coding up the methods. On the other hand, application programmers are concerned only with using that type and calling its methods without worrying much about how the type is implemented.
Classification of data structure:
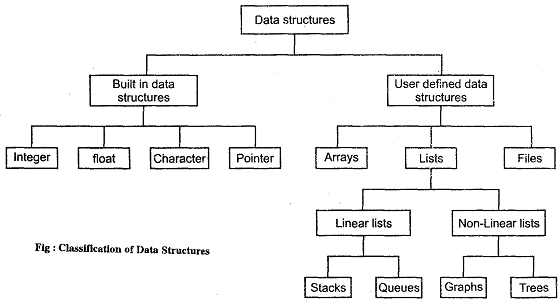
Array
• Array is a group of same type of variables that have common name
• Each item in the group is called an element of the array
• Each element is distinguished from another by an index
• All elements are stored contiguously in memory
• The elements of the array can be of any valid type- integers, characters, floatingpoint types or user-defined types